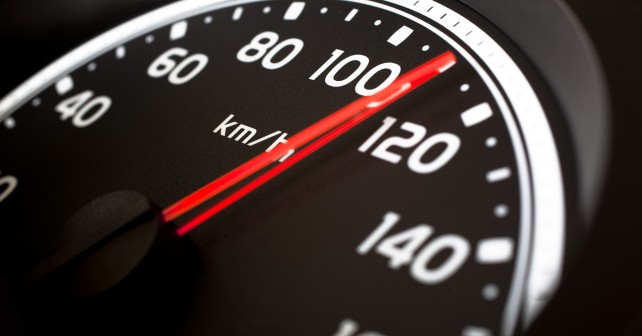
Most of the time, when we buy server infrastructure, we want to verify the download speed of the server. This becomes more critical when we have a proxy server which is downloading images or media from different servers over the internet.
Below is a very simple example to calculate server download speed using Curl in PHP.
php error_reporting(E_ALL | E_STRICT); // Initialize cURL with given url $url = 'http://download.bethere.co.uk/images/61859740_3c0c5dbc30_o.jpg'; $ch = curl_init($url); curl_setopt($ch, CURLOPT_HEADER, 0); curl_setopt($ch, CURLOPT_USERAGENT, "Mozilla/4.0 (compatible; MSIE 6.0; Windows NT 5.1; .NET CLR 1.1.4322)"); curl_setopt($ch, CURLOPT_RETURNTRANSFER, 1); curl_setopt($ch, CURLOPT_CONNECTTIMEOUT, 2); curl_setopt($ch, CURLOPT_TIMEOUT, 60); set_time_limit(65); $execute = curl_exec($ch); $info = curl_getinfo($ch); // Time spent downloading $time = $info['total_time'] - $info['namelookup_time'] - $info['connect_time'] - $info['pretransfer_time'] - $info['starttransfer_time'] - $info['redirect_time']; // Echo friendly messages header('Content-Type: text/plain'); printf("Downloaded %d bytes in %0.4f seconds.\n", $info['size_download'], $time); printf("Which is %0.4f mbps\n", $info['size_download'] * 8 / $time / 1024 / 1024); printf("CURL said %0.4f mbps\n", $info['speed_download'] * 8 / 1024 / 1024); echo "\n\ncurl_getinfo() said:\n", str_repeat('-', 31 + strlen($url)), "\n"; foreach ($info as $label =--> $value){ printf("%-30s %s\n", $label, $value); }
The output will be:
Downloaded 6576848 bytes in 16.2153 seconds.
Which is 3.0944 mbps
CURL said 3.0755 mbps
If you print curl_getinfo()
the output will be:
———————————————————————————————
url http://download.bethere.co.uk/images/61859740_3c0c5dbc30_o.jpg
content_type image/jpeg
http_code 200
header_size 263
request_size 198
filetime -1
ssl_verify_result 0
redirect_count 0
total_time 16.314966
namelookup_time 0.000287
connect_time 0.021524
pretransfer_time 0.021595
size_upload 0
size_download 6576848
speed_download 403117
speed_upload 0
download_content_length 6576848
upload_content_length 0
starttransfer_time 0.056275
redirect_time 0
3 Responses to “Check your server download speed via PHP using CURL”
July 24, 2015
BusinessFirstFamilyI will definitely be reading more if you keep producing posts such as this one.
April 3, 2017
YouAreCopyCatwell this is copied article and code
So many errors . try to be genuine next time
April 4, 2017
Rajinder DeolHi,
it is simple PHP and Curl so nothing fancy or unique. I have tried to use them to check server time. However if you share what errors you have got I will help you more.