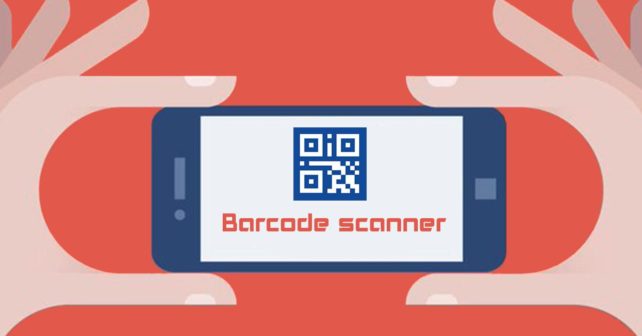
I have written a post about simple Android Barcode Scanner and received few comments about
- How to have the scanner in Vertical layout
- How to turn the camera flash on by default before starting the scan
In this post I will explain these points in detail.
Barcode Scanner in vertical layout
The default implementation of the Zxing library has scanner fixed to “Landscape Mode”. There is no simple way to change that and we have to edit the library files. Zxing library is open source and we can modify the layouts and include it into our project.
After some research and looking into the code on github I found another implementation of Zxing Library. This implementation is not affiliated by the official Zxing guys but the developer did all the changes which we needed for the scanner to open in vertical mode.
This implementation is available as a dependency via jcentre and supports Intents, which makes it a perfect fit for our use case.
Step 1 : Add library as dependency
Create a new project in android studio and add the following code into build.gradle file. We are modifying the application build.gradle file located in your “app” folder
// Added by raj
repositories {
jcenter()
}
// Added by raj ends
dependencies {
compile fileTree(dir: 'libs', include: ['*.jar'])
// Added by raj
compile 'com.journeyapps:zxing-android-embedded:3.4.0'
compile 'com.android.support:appcompat-v7:23.1.0' // Version 23+ is required
// Added by raj ends
}
This code only works for API version 23 and over. Android phones are getting the latest OS releases a lot quicker now days so I have decided not to cover legacy phones.
Step 2 : Create your HomeActivity and layout
I have a simple layout which I have used in my recent posts, we will re-use it again. This layout has a simple “scan” button and two text views to display scanned result.
Following is the code
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent"
android:layout_height="match_parent" android:paddingLeft="@dimen/activity_horizontal_margin"
android:paddingRight="@dimen/activity_horizontal_margin"
android:paddingTop="@dimen/activity_vertical_margin"
android:paddingBottom="@dimen/activity_vertical_margin" tools:context=".HomeActivity">
<TextView
android:text="@string/hello_world"
android:id="@+id/tv_welcome"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginBottom="@dimen/activity_vertical_margin"/>
<TextView
android:text="@string/blog_url"
android:layout_below="@+id/tv_welcome"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:id="@+id/tv_blog_url"
android:layout_marginBottom="@dimen/activity_vertical_margin"/>
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_below="@+id/tv_blog_url"
android:text="@string/btn_scan_now"
android:layout_centerHorizontal="true"
android:onClick="scanNow"
android:layout_marginBottom="@dimen/activity_vertical_margin"
android:id="@+id/btn_scan_now" />
<TextView
android:id="@+id/scan_format"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:textIsSelectable="true"
android:layout_below="@+id/btn_scan_now"
android:gravity="center_horizontal"
android:layout_marginBottom="@dimen/activity_vertical_margin" />
<TextView
android:id="@+id/scan_content"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:textIsSelectable="true"
android:layout_below="@+id/scan_format"
android:layout_marginBottom="@dimen/activity_vertical_margin"
android:gravity="center_horizontal" />
</RelativeLayout>
In the HomeActivity.java create a function named “scanNow” this function is attached to the onClick event of the scan button in our layout.
public void scanNow(View view){
IntentIntegrator integrator = new IntentIntegrator(this);
integrator.setDesiredBarcodeFormats(IntentIntegrator.ONE_D_CODE_TYPES);
integrator.setPrompt(this.getString(R.string.scan_bar_code));
integrator.setCameraId(0); // Use a specific camera of the device
integrator.setBarcodeImageEnabled(true);
integrator.initiateScan();
}
This function will use Intent and launch the scanner. Scanner requires “onActivityResult” function defined in the calling Activity. The result of scan will be passed to this function.
public void onActivityResult(int requestCode, int resultCode, Intent intent) {
//retrieve scan result
IntentResult scanningResult = IntentIntegrator.parseActivityResult(requestCode, resultCode, intent);
if (scanningResult != null) {
if(scanningResult.getContents() != null){
//we have a result
codeContent = scanningResult.getContents();
codeFormat = scanningResult.getFormatName();
// display it on screen
formatTxt.setText("FORMAT: " + codeFormat);
contentTxt.setText("CONTENT: " + codeContent);
}else{
Toast.makeText(this, "Cancelled", Toast.LENGTH_LONG).show();
}
}else{
Toast toast = Toast.makeText(getApplicationContext(),"No scan data received!", Toast.LENGTH_SHORT);
toast.show();
}
}
The library has CaptureActivity.java which will be called by the intent to launch the scanner. To display the scanner in Vertical layout we need to set the orientation of CaptureActivity to “fullSensor”, but instead of editing the library files we will create a new activity which will extend CaptureActivity and add orientation to it.
Create a new activity named “AnyOrientationCaptureActivity” which extends CaptureActivity
package in.whomeninja.android_barcode_scanner_bulk_scan_with_flash;
import com.journeyapps.barcodescanner.CaptureActivity;
/**
* This Activity is exactly the same as CaptureActivity, but has a different orientation
* setting in AndroidManifest.xml.
* Created by RajinderPal on 1/29/2017.
*/
public class AnyOrientationCaptureActivity extends CaptureActivity {
}
Now add the Activity defination in AndroidManifest.xml with orientation as “fullSensor”
<activity android:name=".AnyOrientationCaptureActivity"
android:screenOrientation="fullSensor"
android:stateNotNeeded="true"
android:theme="@style/zxing_CaptureTheme"
android:windowSoftInputMode="stateAlwaysHidden">
</activity>
We have to update the Intent integrator to use our Activity as CaptureActivity. Add the following lines to “scanNow” function.
integrator.setCaptureActivity(AnyOrientationCaptureActivity.class);
integrator.setOrientationLocked(false);
Compile and build your project. You will see the scanner in vertical orientation.
Turn the flash on by default on scan
Turning the flash on and off is already added in the library by using volume up and volume down buttons. Volume up button will turn the camera flash on and volume down will turn the camera flash off. However there is no support for the default state of camera flash in the intent.
In this library we can specify the capture activity via intent and it will allow us to define our custom activity and do the changes that we need. Create a new activity by the name TorchOnCaptureActivity and copy the content of library’s Capture activity in it. Library’s Capture activity is located at com.journeyapps.barcodescanner.CaptureActivity
The source code for this file is located on my github at TorchOnCaptureActivity. We have to make the changes in the onCreate method so I have decided to create a new activity instead of extending the original CaptureActivity.
The scanner view already has a function called setTorchOn which is used in event handling of volume up button. We will use the same function and call it immediately after initializing the view in onCreate method.
Now the onCretae function should look like
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
barcodeScannerView = initializeContent();
// turn the flash ON by default
barcodeScannerView.setTorchOn();
capture = new CaptureManager(this, barcodeScannerView);
capture.initializeFromIntent(getIntent(), savedInstanceState);
capture.decode();
}
Now add the Activity defination in AndroidManifest.xml
<activity android:name=".TorchOnCaptureActivity"
android:screenOrientation="fullSensor"
android:stateNotNeeded="true"
android:theme="@style/zxing_CaptureTheme"
android:windowSoftInputMode="stateAlwaysHidden">
</activity>
If you notice I have kept the screenOrientation
as fullSensor
for vertical orientation. Now go to the HomeActivity and change the scanNow function. Update
setCaptureActivity
and setOrientationLocked
as :
integrator.setCaptureActivity(TorchOnCaptureActivity.class);
integrator.setOrientationLocked(false);
Compile and build your project and now camera flash should be on by default.
However we have to make it configurable, so that we can decide from our intent if we want the camera flash on by default. Intent has a method called addExtra
which we can use to add any additional data to the intent and receive the same at the activity which intent is starting.
We will add a boolean flag to the intent to set camera flash on. We need to provide a key name for this flag so that we can use the same name to access the data at receiving activity. As a best practice all your constants should be defined in a separate class. This will reduce the amount of code change if you ever have to change the key name.
Create a class named appConstants and define a constant CAMERA_FLASH_ON
package in.whomeninja.android_barcode_scanner_bulk_scan_with_flash;
/**
* Created by rajinders on 15/05/17.
*/
public final class appConstants {
public static final String CAMERA_FLASH_ON = "CAMERA_FLASH_ON";
}
We will use this constant to send flag for turning the flash On. Modify the scanNow
function in HomeActivity and add
integrator.addExtra(appConstants.CAMERA_FLASH_ON,true);
We will now modify our TorchOnCaptureActivity class to check for this flag and turn the flash on if the flag it set to boolean true. The onCreate function of TorchOnCaptureActivity class will now look like:
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
barcodeScannerView = initializeContent();
// turn the flash on if set via intent
Intent scanIntent = getIntent();
if(scanIntent.hasExtra(appConstants.CAMERA_FLASH_ON)){
if(scanIntent.getBooleanExtra(appConstants.CAMERA_FLASH_ON,false)){
barcodeScannerView.setTorchOn();
updateView();
}
}
capture = new CaptureManager(this, barcodeScannerView);
capture.initializeFromIntent(getIntent(), savedInstanceState);
capture.decode();
}
Add Flash On and Off button
Although by default volume-up and volume-down button of the phone can turn the camera flash on and off but it will be more user friendly if we have a button on the scan screen to turn the camera flash on and off.
To add a button on the scan screen we have to create a custom layout which will have our scan area and additional buttons. This library provides DecoratedBarcodeView which we can add to our custom layout to bring the barcode scanner. Lets create a new layout file named capture_flash in the res folder. This layout will have two buttons to turn the camera flash on and off. We will also add com.journeyapps.barcodescanner.DecoratedBarcodeView to bring the scanner screen.
The layout capture_flash.xml will have the following code:
<?xml version="1.0" encoding="UTF-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
xmlns:app="http://schemas.android.com/apk/res-auto"
android:orientation="vertical"
android:layout_width="match_parent"
android:layout_height="match_parent">
<Button
android:id="@+id/switch_flashlight_on"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="@string/turn_on_flashlight"
android:layout_alignParentTop="true"
android:layout_centerHorizontal="true"
android:visibility="visible"
android:onClick="toggleFlash"/>
<Button
android:id="@+id/switch_flashlight_off"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="@string/turn_off_flashlight"
android:layout_alignParentTop="true"
android:layout_centerHorizontal="true"
android:visibility="gone"
android:onClick="toggleFlash"/>
<com.journeyapps.barcodescanner.DecoratedBarcodeView
android:layout_alignParentLeft="true"
android:layout_alignParentRight="true"
android:layout_alignParentBottom="true"
android:layout_alignParentTop="true"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:layout_marginLeft="50dp"
android:layout_marginRight="50dp"
android:layout_marginTop="150dp"
android:layout_marginBottom="150dp"
android:id="@+id/zxing_barcode_scanner"
app:zxing_use_texture_view="false"
app:zxing_preview_scaling_strategy="fitXY"/>
</RelativeLayout>
Update the initializeContent function in TorchOnCaptureActivity to use this layout.
protected DecoratedBarcodeView initializeContent() {
setContentView(R.layout.capture_flash);
return (DecoratedBarcodeView)findViewById(com.google.zxing.client.android.R.id.zxing_barcode_scanner);
}
We have to create a function named toggleFlash to handle click event of the buttons. Also we have to create a variable named cameraFlashOn to hold the current state of camera flash. Add a private variable in TorchOnCaptureActivity:
private boolean cameraFlashOn = false;
Based on the value of this variable we will turn the camera flash on and off. The toggleFlash function in TorchOnCaptureActivity will have the following code:
public void toggleFlash(View view){
if(cameraFlashOn){
barcodeScannerView.setTorchOff();
}else{
barcodeScannerView.setTorchOn();
}
}
The DecoratedBarcodeView provides TorchListener which is being called every time when camera flash is turned on or off. We will create a listener class by implementing DecoratedBarcodeView.TorchListener interface with two methods onTorchOn and onTorchOff. This listener class will take the context of our activity TorchOnCaptureActivity and update the value of cameraFlashOn variable. We will create the listener class within the TorchOnCaptureActivity class.
class TorchEventListener implements DecoratedBarcodeView.TorchListener{
private TorchOnCaptureActivity activity;
TorchEventListener(TorchOnCaptureActivity activity){
this.activity = activity;
}
@Override
public void onTorchOn() {
this.activity.cameraFlashOn = true;
this.activity.updateView();
}
@Override
public void onTorchOff() {
this.activity.cameraFlashOn = false;
this.activity.updateView();
}
}
We have to create updateView function to show or hide flash-on and flash-off buttons whenever the flash state is changed. The code for updateView function will be:
public void updateView(){
if(cameraFlashOn){
turnflashOn.setVisibility(View.GONE);
turnflashOff.setVisibility(View.VISIBLE);
}else{
turnflashOn.setVisibility(View.VISIBLE);
turnflashOff.setVisibility(View.GONE);
}
}
Now we have to initialise our listener and attach it to the barcodeScannerView. This will be done in the onCreate function of TorchOnCaptureActivity. The updated onCreate function will have the following code:
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
barcodeScannerView = initializeContent();
TorchEventListener torchEventListener = new TorchEventListener(this);
barcodeScannerView.setTorchListener(torchEventListener);
turnflashOn = findViewById(R.id.switch_flashlight_on);
turnflashOff = findViewById(R.id.switch_flashlight_off);
// turn the flash on if set via intent
Intent scanIntent = getIntent();
if(scanIntent.hasExtra(appConstants.CAMERA_FLASH_ON)){
if(scanIntent.getBooleanExtra(appConstants.CAMERA_FLASH_ON,false)){
barcodeScannerView.setTorchOn();
updateView();
}
}
capture = new CaptureManager(this, barcodeScannerView);
capture.initializeFromIntent(getIntent(), savedInstanceState);
capture.decode();
}
Compile and build your code and now you will have flash-on button on the scan screen.
This listener implementation will update the flash-on and flash-off buttons even when camera flash is turned on or off from volume-up and volume-down buttons.
Full source code of the project can be found at my github under android-barcode-scanner-bulk-scan-with-flash
49 Responses to “Android Barcode Scanner vertical orientation (portrait mode) and camera flash”
March 20, 2017
kayaceThanks for teaching. I have a question. How can I do half of layout scanning and other part showing the TextView, ScanButton etc. ?
Have a nice day.
February 1, 2018
AlankritaHave you found how to do it?
February 2, 2018
Rajinder DeolHi Alankrita,
this can be done in the same way I have added flash ON and OFF button on the scan screen, if that doesn’t work for you, can you share some more detail of what you have tried.
April 5, 2017
VidyaHi Raj..
Great tutorial.
I have question how to modify rectangle of scanning bar- code means in UI??
April 6, 2017
Rajinder DeolHi Vidya,
thanks for your kind words,What do you want to change on the UI ?. You can control the full UI via BarcodeView and DecoratedBarcodeView. If you tell me what do you want to achieve I can point you in right direction.
April 6, 2017
VidyaHi Raj,
Thanks for Reply 🙂
As u told in post we can On torch using Volume Up button and off using volume Off button of our mobile.
but if user is new and he don’t know an=bout this things so i just want to provide on button on screen like flashlight so user can On/Off using it.
so i want to know is it good or not? if yes then how to implement it?
I have on more question
I am able to scan Bar-code. but in my project there is requirement to scan only one type of product for which we are implementing this .so we want to check is scan bar-code is of right product if yes then want to pair that product.
so how to do it?
need help
April 7, 2017
Rajinder DeolHi Vidya,
well it is a good idea to have both volume buttons and button on screen to control the flash light. I will update the post to add the button on screen as well.
About the “product pair” I don’t understand your question.
April 7, 2017
VidyaHi Raj,
Actually i ma developing this barcode scan app for tire.so currently i am able to scan all types of products. so is it possible to check online scanned product is right using barcode? or is there any site to check barcode online using barcode number?
April 7, 2017
Rajinder DeolHey Vidya,
barcode will provide you the barcode string you need to have a database to search the product details. Depending upon your product it can be your personal database or any third party service. I don,t know any service or API as such but you can search on google.
April 7, 2017
Vidyayes i get result in the form of
Barcode type like EAN or UPC and barcode number.
so I have to store it in database? which database will be good sqlite or other than sqlite like couchbase?
and Is every product has their own barcode database?
April 10, 2017
Rajinder DeolHi Vidya,
you can select any database based on your requirement, sqllite will be good to start with. If your application size is huge then you can use couchbase.
April 10, 2017
vidyaHi Raj ,
Sometimes i am not able to scan some barcode like
https://worldbarcodes.com/wp-content/uploads/39123439-code39.gif
why this is happened? can u please help help me regarding this?
April 10, 2017
Rajinder DeolHi Vidya,
the library only supports Barcode formats : “UPC_A”, “UPC_E”, “EAN_8”, “EAN_13”, “CODE_39”, “CODE_93”, “CODE_128”
Make sure your barcode is in these formats
April 10, 2017
vidyaOkay Raj.
Can we use both QR and Barcode together???
April 10, 2017
vidyameans without using fragment?
integrator.setDesiredBarcodeFormats(IntentIntegrator.ONE_D_CODE_TYPES );
using above statement i am bale to scan only 1D barcode like below format but i want to scan QR code also.
any suggestion for this?
mobile vision is good option or zxing?
public static final Collection ONE_D_CODE_TYPES =
list(“UPC_A”, “UPC_E”, “EAN_8”, “EAN_13”, “CODE_39”, “CODE_93”, “CODE_128”,
“ITF”, “RSS_14”, “RSS_EXPANDED”);
April 11, 2017
Rajinder DeolHi Vidya,
you can scan Barcodes and QR-codes but one at a time, you need to have two buttons one for Barcode and one for QR-code and based on the button pressed you have to set for QR-code :
integrator.setDesiredBarcodeFormats(IntentIntegrator.QR_CODE_TYPES);
and for Barcode :
integrator.setDesiredBarcodeFormats(IntentIntegrator.ONE_D_CODE_TYPES);
April 11, 2017
vidyaOkay Raj… I check mobile vision. which scan both QR and BAR code using single button so i thought can we do this thing with zxing?
April 11, 2017
Rajinder Deolzxing is the last mile to which you supply the image part, mobile vision detects the whole image and then grab the image portion. zxing is a very simple implementation.
April 17, 2017
DeboHi Raj,
Im usuing your this tutorial in my project.Im new in android devlopment.Can u suggest me some tutorial on
“How to retrieve data from remote database against QR-code value and display automatically in the app.”
http://stackoverflow.com/questions/43443516/how-to-retrieve-data-from-remote-database-against-qr-code-value-and-display-auto
April 18, 2017
Rajinder DeolHi Debo,
you can use PHP and MYSQL in the backend and create a REST API which your app can call and fetch the information. Here is a quick tutorial : https://www.programmableweb.com/news/how-to-build-restful-apis-using-php-and-laravel/how-to/2014/08/13
you can then follow my post Writing Secure REST webservices with PHP for Android to call the API
April 25, 2017
BrianHi Raj
I have integrated knowledge gained from the tutorial for barcode scanner.
I would like to know how to validate the read data. Like if a person rescans the barcode , it informs he or she that the code is already scanned
April 26, 2017
Rajinder DeolHi Brain,
you need to save the scanned data in local storage and compare the scanned barcode with already stored data. You can read Android Aadhaar Card Scanner to get an idea on how to save and read data from local storage.
Let me know if you need more help.
May 5, 2017
vidyaHi raj,
If I want to change Barcode Scanner Screen then I have to used custom layout???
May 21, 2017
Rajinder DeolHi Vidya,
yes, you have to use a custom layout. I have implemented this in Android Barcode Scanner vertical orientation (portrait mode) and camera flash
August 18, 2017
ManuelHola Rajinder Deol, felicitarte por tu excelente tutorial.
Como podría escanear el código de barras que esta de forma vertical?
August 18, 2017
Rajinder DeolHi Manuel,
the app should be able to scan vertical barcodes as well, if you have tried and it didn’t work, can you please share an example
September 29, 2017
VorngHi Rajinder Deol,
Thanks for your great tutorial.I am newly with android. I can scan QR code by as you show integrator.setDesiredBarcodeFormats(IntentIntegrator.QR_CODE_TYPES);
When QR code generate as (Link of url),how can we open webpage automatically after scan QR code?
Thanks you.
October 3, 2017
Rajinder DeolHi maotorngvorng49,
you need to validate the scanned text if it is a valid URL and then open it in a webview. I have created a version of Homeactivity.java for you with the validation and opening in webview code. check it here open scanned QR-Code URL in webview
you have to add a webview in your application layout to open the URL.
October 6, 2017
vorngThanks you. But I have an error when run with emulator.
October 7, 2017
Rajinder DeolThis will not work with emulator as it requires camera access, you need to test it on a real device
October 18, 2017
vorngThanks you.I have removed ScanNow button and call it directly. Could you have me how to continue scan.Many thanks.
October 19, 2017
Rajinder DeolYou can do a continuous scan to keep on scanning, I will write a post over the weekend with details.
October 14, 2017
beginnerThank for great tutorial.
November 18, 2017
Dhananjay SInghhello sir ,
I tried to set orientation to “portrait” of scanner camera by the use of “IntentIntegrator” class method below….
IntentIntegrator intentManager=new IntentManager();
intentManager.setOrientationLocked(false);
intentManager.setCaptureActivity(CaptureActivity.class);
But it’s not working please suggest other option to set it portrait.
November 19, 2017
Rajinder DeolHi Dhananjay,
are you using the library mentioned in the post, this change will not work with official zxing library.
November 21, 2017
Dhananjay SInghHello sir,
Thankyou for reply,
Yes sir i am using zxing library.
My issue was resolved.
—————————————-
I want to know that..?
“can i manage an image-icon for flash light on and off, after open the camera or scanner.”
If yes how can i resolve it.
November 21, 2017
Rajinder DeolHi Dhananjay,
good to know that you have solved the error, if you go through the post I have added two buttons to turn the flash on and off. You can use
ImageButton
instead ofButton
and update the onclick function.For more details on imagebuttons read the docs here
November 21, 2017
Dhananjay SInghHello Sir,
Thank you sir.
Problem resolved.
Sir can i scan only mobile number from any image or from any id card…?
Please give me some suggestion.
November 21, 2017
Dhananjay SInghHello Sir
Thankyou,i resolved image problem.
Sir i want know that i can scan only mobile number from id card and images ,please suggest me.
November 22, 2017
Rajinder DeolHey Dhananjay,
I did not understand your question, what are you trying to scan? Barcode can only have numbers and letters, however QRcode can have any string.
November 23, 2017
Dhananjay SInghHello Sir ,
I want to say that i want to scan only contact “Number” from any id card or any Image or poster.
“But not all text only digits of contact number.”
December 9, 2017
Rajinder DeolHi Dhananjay,
you need Optical Character Recognition (OCR) functionality, there are people who have tried it already you can read
http://roncemer.com/software-development/java-ocr/
https://stackoverflow.com/questions/36453199/offline-image-to-text-recognition-ocr-in-android
https://github.com/renard314/textfairy
https://github.com/testica/text-scanner
June 8, 2019
ArsalanSir Its a great tutorail.
How to increase the scanning speed because its takes too mauch time to scan barcode and qrcode.
June 23, 2019
Rajinder DeolHi Arsalan,
the code uses image captured by the phone camera, so the speed of scan depends on the quality of image captured by the camera. Can you try the code on a different phone with better camera and let me know if you face the same issue.
October 12, 2019
premHi rajendra,
can we scan both type code while using this
integrator.setDesiredBarcodeFormats(IntentIntegrator.ALL_CODE_TYPES); ?
March 17, 2020
Rajinder DeolHi Prem,
if you are asking for QR code as well as Barcode then no, this library doesn’t recognise images automatically.
April 9, 2020
Luis BordinoHello, I am new to programming in android studio. I have programmed in AppInventor a scanner app to read an ad/hoc generated qr code (one per box) to include units and kilograms in a box.
But it takes forever to scan because it reloads the scanner for each new scan (up to 5 seconds per cycle).
How can I implement this code in android studio? is this Java or Kotlin? or none? Thanks!!!!
April 9, 2020
Rajinder DeolHi Luis,
this code is java based and the project is android studio project, you can clone my repository and import it into android studio.
October 25, 2022
Akash JadhavHello sir,
when i use this library with navigation component in android so back button not working
navigation.popUpBackStack() not working
navigation.navigateUp() also not working